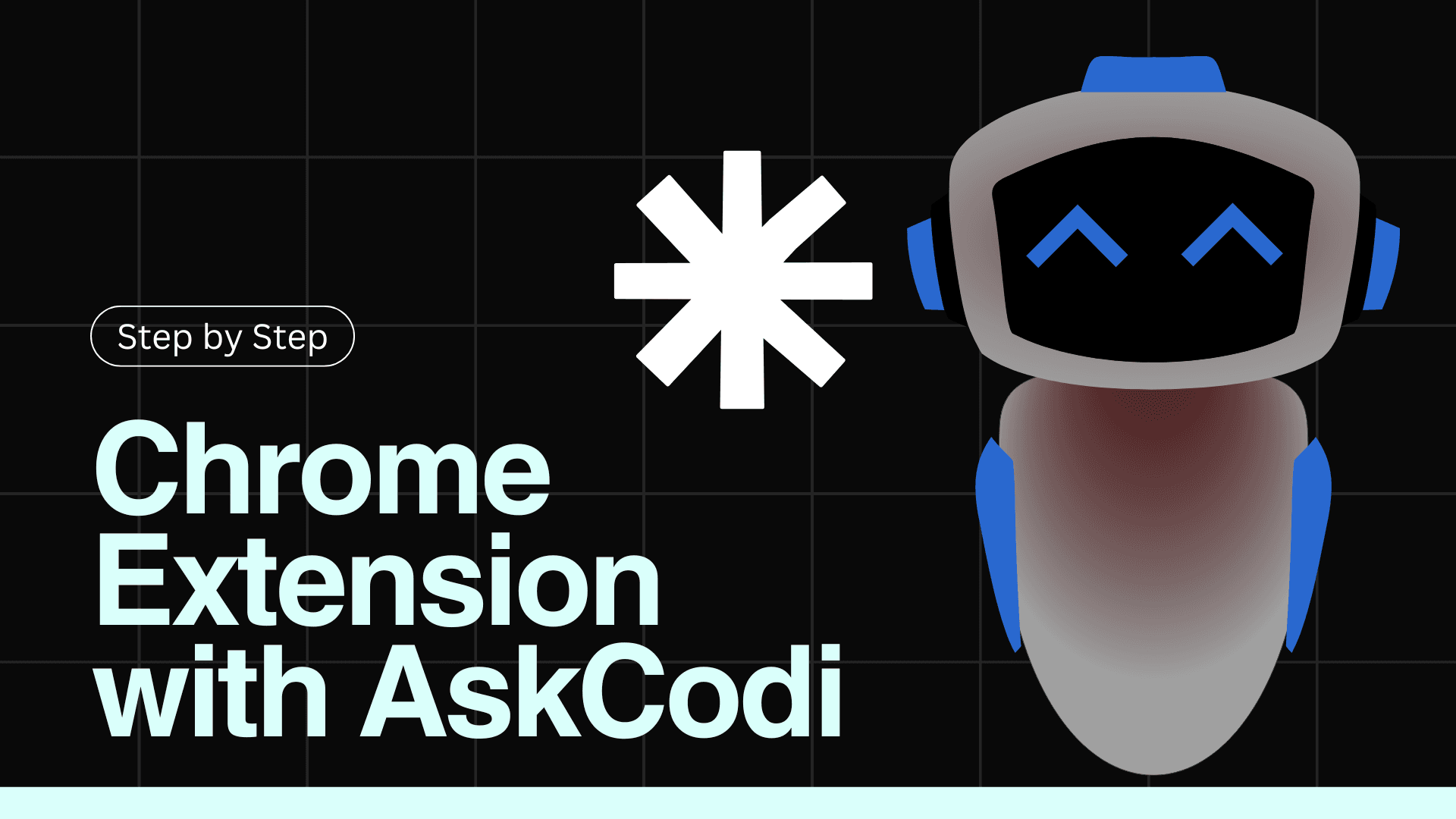
Building Your First Chrome Extension with AskCodi: A Step-by-Step Guide
Sachin Sharma
5/2/2025
As a developer, I've always been fascinated by browser extensions. They're like little magic wands that can transform our browsing experience in countless ways. Today, I'm excited to share my journey of creating a Chrome extension using AskCodi, an AI-powered coding assistant, right within Visual Studio Code (VS Code).
Whether you're a seasoned developer or just starting out, this guide will walk you through the process, making it accessible and fun. So, let's roll up our sleeves and dive in!
Prerequisites
Before we embark on this coding adventure, make sure you have:
- Google Chrome installed (obviously!)
- Visual Studio Code as your code editor
- AskCodi extension installed in VS Code
- Basic knowledge of HTML, CSS, and JavaScript
Step 1: Setting up the VS Code Environment with AskCodi
Let’s start by ensuring your environment is ready.
-
Open VS Code and make sure you have installed the AskCodi extension. If you haven't yet:
- Head to the Extensions tab (or use
Ctrl+Shift+X
). - Search for AskCodi.
- Click Install.
- Head to the Extensions tab (or use
-
You’ll also need API key for AskCodi which you can get in your account settings at https://askcodi.com. You can follow the detailed instrunctions in AskCodi docs
-
You can choose between a range of models, I'm going with Claude 3.5 sonnet.
Here's a friendly little screenshot of how it looks when you’ve got AskCodi installed:
Step 2: Setting Up Your Project
Let's start by creating a cozy home for our extension:
-
Create a new folder on your computer, for example,
my-chrome-extension
. Inside this folder, open VS Code. -
Alternatively, you can also use the terminal. Open your terminal and create a new directory and open VS Code:
mkdir MyChromeExtension cd MyChromeExtension code .
- Now, let's create the essential files for our extension:
- manifest.json
- popup.html
- content.js
- popup.js
- styles.css
You can do this manually or use the VS Code file explorer. I prefer the latter - it's just so satisfying to click that 'New File' button!
Step 3: Crafting the Manifest
The manifest.json file is like the ID card of your extension. It tells Chrome what your extension is all about. Let's use AskCodi to help us write this file:
- Open manifest.json in VS Code.
- Open AskCodi panel from right sidebar of VS Code to summon our AI assistant.
- Ask: "Help me write a basic manifest.json for a Chrome extension that changes the background color of a webpage."
AskCodi should provide something like below code. Notice AskCodi suggests to add icon images in images directory. So go ahead, create the images directory and add icon pngs.
{ "manifest_version": 3, "name": "Background Color Changer", "version": "1.0", "description": "A simple extension to change the background color of webpages.", "permissions": ["activeTab"], "action": { "default_popup": "popup.html", "default_icon": { "16": "images/icon16.png", "48": "images/icon48.png", "128": "images/icon128.png" } }, "icons": { "16": "images/icon16.png", "48": "images/icon48.png", "128": "images/icon128.png" }, "content_scripts": [ { "matches": ["<all_urls>"], "js": ["content.js"] } ] }
Step 4: Creating the Popup
Now, let's create a simple popup for our extension:
Open popup.html and ask AskCodi: "Write HTML for a popup with a color picker input and a 'Change Color' button with styling in styles.css"
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Background Color Changer</title> <link rel="stylesheet" href="styles.css"> </head> <body> <div class="container"> <h1>Background Color Changer</h1> <div class="color-picker"> <label for="colorInput">Choose a color:</label> <input type="color" id="colorInput" value="#ffffff"> </div> <button id="changeColorBtn">Change Color</button> </div> <script src="popup.js"></script> </body> </html>
For styles.css, let's make it look nice:
body { font-family: Arial, sans-serif; width: 300px; padding: 10px; background-color: #f0f0f0; } .container { display: flex; flex-direction: column; align-items: center; } h1 { color: #333; font-size: 18px; margin-bottom: 15px; } .color-picker { margin-bottom: 15px; display: flex; flex-direction: column; align-items: center; } label { margin-bottom: 5px; color: #555; } #colorInput { width: 100px; height: 40px; border: none; cursor: pointer; } #changeColorBtn { background-color: #4CAF50; border: none; color: white; padding: 10px 20px; text-align: center; text-decoration: none; display: inline-block; font-size: 16px; margin: 4px 2px; cursor: pointer; border-radius: 4px; transition: background-color 0.3s; } #changeColorBtn:hover { background-color: #45a049; }
In my case, AskCodi also suggested changes in manifest.json to include the styles file. here is updated manifest.json:
{ "manifest_version": 3, "name": "Background Color Changer", "version": "1.0", "description": "A simple extension to change the background color of webpages.", "permissions": [ "activeTab" ], "action": { "default_popup": "popup.html", "default_icon": { "16": "images/icon16.png", "48": "images/icon48.png", "128": "images/icon128.png" } }, "web_accessible_resources": [ { "resources": [ "styles.css" ], "matches": [ "<all_urls>" ] } ], "icons": { "16": "images/icon16.png", "48": "images/icon48.png", "128": "images/icon128.png" }, "content_scripts": [ { "matches": [ "<all_urls>" ], "js": [ "content.js" ] } ] }
Step 5: Adding Functionality
Now for the fun part - making our extension do something! Open popup.js and let's ask AskCodi for help: "Write JavaScript to change the background color of the current tab when the 'Change Color' button is clicked" AskCodi should provide something like below:
- For content.js:
chrome.runtime.onMessage.addListener(function(request, sender, sendResponse) { if (request.action === "changeColor") { document.body.style.backgroundColor = request.color; } });
- For popup.js:
document.addEventListener('DOMContentLoaded', function() { var changeColorBtn = document.getElementById('changeColorBtn'); var colorInput = document.getElementById('colorInput'); changeColorBtn.addEventListener('click', function() { var color = colorInput.value; chrome.tabs.query({active: true, currentWindow: true}, function(tabs) { chrome.tabs.sendMessage(tabs[0].id, {action: "changeColor", color: color}); }); }); });
Step 6: Testing Your Extension
Now comes the moment of truth! Let's load our extension into Chrome:
- Open Chrome and navigate to chrome://extensions/
- Enable "Developer mode" in the top right
- Click "Load unpacked" and select your extension directory
You should see your extension icon appear in the Chrome toolbar. Click it, choose a color, and voilà! Watch the magic happen as you transform web pages with a click.
Wrapping Up
And there you have it! Your very own Chrome extension, brought to life with the help of AskCodi. We've only scratched the surface of what's possible with extensions, but I hope this guide has sparked your curiosity to explore further.
Remember, the key to becoming a great developer is experimentation. Don't be afraid to tweak the code, add new features, or even start a completely new project. The possibilities are endless! Happy coding, and may your browsing experience never be the same again! 🚀🎨
See why AskCodi beats other AI assistants every time.
Discover all the amazing things you'll create with AI‑powered coding assistance.