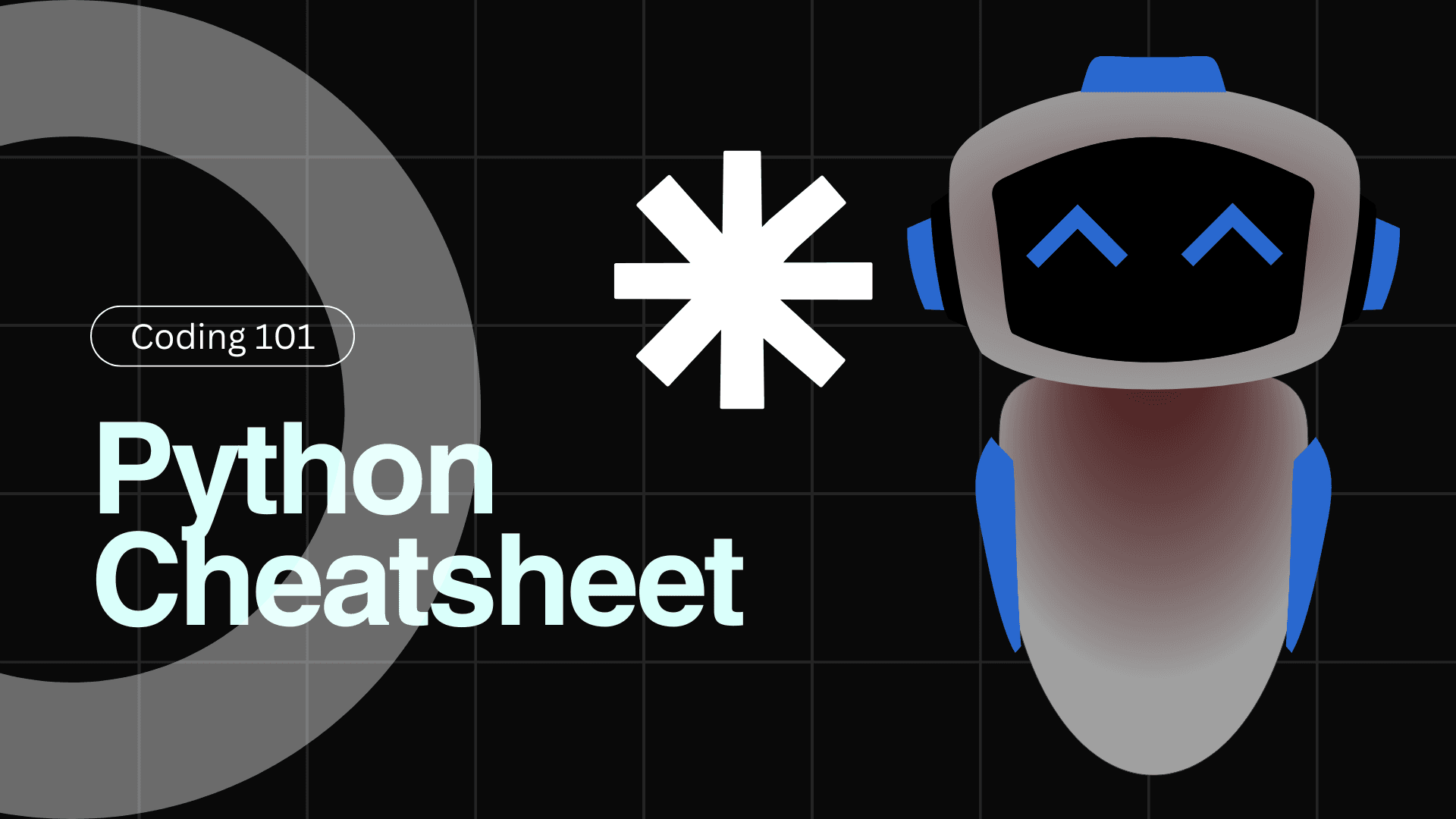
Python Cheatsheet: Comprehensive Overview
S
Sachin Sharma
4/19/2025
1. Introduction to Python
What is Python?
- Python is a high-level, interpreted programming language known for its readability and simplicity.
- Created by Guido van Rossum and first released in 1991.
- Emphasizes code readability with its use of significant indentation.
Why Learn Python?
- Widely used in web development, data science, machine learning, automation, and more.
- Large standard library and active community support.
- Platform-independent and open-source.
Installing Python
- Download from: python.org/downloads
- Verify installation:
orpython --version
python3 --version
Setting Up the Development Environment
- IDEs: PyCharm, Visual Studio Code, Sublime Text, Atom.
- Command Line: Use terminal or command prompt with a text editor.
- Virtual Environments:
python -m venv env source env/bin/activate # On Windows: env\Scripts\activate
2. Python Basics
Syntax and Semantics
- Indentation: 4 spaces per indent level.
if condition: # indented block execute_code()
- Comments:
# This is a single-line comment
Variables and Data Types
- Variables: No explicit declaration required.
x = 10 # Integer y = 3.14 # Float name = "Alice" # String
- Data Types:
int
,float
,str
,bool
,list
,tuple
,dict
,set
, etc.
Operators
- Arithmetic:
+
,-
,*
,/
,%
(modulus),**
(exponent),//
(floor division). - Assignment:
=
,+=
,-=
,*=
,/=
, etc. - Comparison:
==
,!=
,>
,<
,>=
,<=
. - Logical:
and
,or
,not
. - Bitwise:
&
,|
,^
,~
,<<
,>>
.
Input and Output
- Input:
user_input = input("Enter something: ")
- Output:
print("Hello, World!")
3. Control Flow Statements
Conditional Statements
if condition: # code block elif another_condition: # another code block else: # else code block
Loops
- For Loops:
for i in range(5): print(i)
- While Loops:
while condition: # code block
Loop Control Statements
- break: Exits the loop.
for i in range(10): if i == 5: break
- continue: Skips to the next iteration.
for i in range(10): if i % 2 == 0: continue print(i)
- pass: Null operation; placeholder.
if condition: pass
4. Functions
Defining Functions
def function_name(parameters): """Docstring""" # code block return value
Example:
def add(a, b): return a + b
Lambda Functions
square = lambda x: x ** 2 print(square(5)) # Output: 25
Variable Scope
- Local: Defined within a function.
- Global: Defined outside all functions.
global_var = "I am global" def foo(): local_var = "I am local"
Recursion
def factorial(n): if n == 1: return 1 else: return n * factorial(n - 1)
5. Data Structures
Lists
- Creation:
numbers = [1, 2, 3, 4, 5]
- Indexing/Slicing:
first = numbers[0] slice = numbers[1:3]
- Methods:
numbers.append(6) numbers.remove(2) numbers.sort()
- List Comprehensions:
squares = [x ** 2 for x in range(10)]
Tuples
- Creation:
point = (1, 2)
- Accessing Elements:
x = point[0]
Dictionaries
- Creation:
person = {'name': 'Alice', 'age': 30}
- Accessing Values:
name = person['name']
- Methods:
person.keys() person.values() person.items()
Sets
- Creation:
unique_numbers = {1, 2, 3}
- Operations:
set1.union(set2) set1.intersection(set2) set1.difference(set2)
6. Modules and Packages
Importing Modules
import math print(math.pi)
From Import
from math import sqrt print(sqrt(16))
Third-Party Packages
- Installing:
pip install requests
- Using:
import requests response = requests.get('https://api.example.com')
Creating Modules
- my_module.py
def greet(name): return f"Hello, {name}!"
- Using the Module:
from my_module import greet print(greet("Bob"))
7. Object-Oriented Programming (OOP)
Classes and Objects
class Person: def __init__(self, name): self.name = name def greet(self): print(f"Hello, my name is {self.name}") p = Person("Alice") p.greet()
Inheritance
class Employee(Person): def __init__(self, name, employee_id): super().__init__(name) self.employee_id = employee_id
Encapsulation
- Private Attributes (conventionally):
class MyClass: def __init__(self): self.__private_var = 42
Polymorphism
- Method Overriding:
class Animal: def speak(self): pass class Dog(Animal): def speak(self): print("Woof!")
Abstraction
- Abstract Classes (using
abc
module):from abc import ABC, abstractmethod class Shape(ABC): @abstractmethod def area(self): pass
8. File Handling
Opening Files
file = open('filename.txt', 'r') # Modes: r, w, a, rb, wb, etc.
Reading Files
content = file.read() lines = file.readlines()
Writing to Files
file = open('filename.txt', 'w') file.write("Hello, World!")
Closing Files
file.close()
Using Context Managers
with open('filename.txt', 'r') as file: content = file.read() # File is automatically closed here
9. Exception Handling
Try...Except Blocks
try: # code that may raise an exception result = 10 / 0 except ZeroDivisionError: print("Cannot divide by zero")
Else and Finally
try: result = 10 / 2 except ZeroDivisionError: print("Cannot divide by zero") else: print("Result is", result) finally: print("Execution completed")
Raising Exceptions
def check_age(age): if age < 0: raise ValueError("Age cannot be negative")
10. Advanced Topics
Iterators and Generators
-
Iterator Protocol:
iter_obj = iter([1, 2, 3]) print(next(iter_obj))
-
Generators:
def my_generator(): yield 1 yield 2 yield 3 for value in my_generator(): print(value)
Decorators
def decorator_function(original_function): def wrapper_function(*args, **kwargs): # Do something before result = original_function(*args, **kwargs) # Do something after return result return wrapper_function @decorator_function def display(): print("Display function ran")
Multithreading and Multiprocessing
- Multithreading:
import threading def print_numbers(): for i in range(5): print(i) thread = threading.Thread(target=print_numbers) thread.start()
- Multiprocessing:
from multiprocessing import Process def print_numbers(): for i in range(5): print(i) process = Process(target=print_numbers) process.start()
Regular Expressions
import re pattern = r'\d+' # Matches one or more digits text = "There are 12 apples" matches = re.findall(pattern, text) print(matches) # Output: ['12']
11. Working with Data
NumPy for Numerical Computing
import numpy as np array = np.array([1, 2, 3]) print(array * 2) # Output: [2, 4, 6]
Pandas for Data Analysis
import pandas as pd data = {'Name': ['Alice', 'Bob'], 'Age': [30, 25]} df = pd.DataFrame(data) print(df)
Matplotlib for Visualization
import matplotlib.pyplot as plt plt.plot([1, 2, 3], [4, 9, 16]) plt.xlabel('X Axis') plt.ylabel('Y Axis') plt.title('Sample Plot') plt.show()
12. Web Development
Flask Example
from flask import Flask app = Flask(__name__) @app.route('/') def home(): return "Hello, Flask!" if __name__ == '__main__': app.run()
Django Example
- Install Django:
pip install django
- Create Project:
django-admin startproject myproject
- Run Server:
python manage.py runserver
13. Database Interaction
SQLite with sqlite3 Module
import sqlite3 conn = sqlite3.connect('example.db') cursor = conn.cursor() cursor.execute('CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, name TEXT)') cursor.execute('INSERT INTO users (name) VALUES (?)', ('Alice',)) conn.commit() cursor.execute('SELECT * FROM users') print(cursor.fetchall()) conn.close()
Using SQLAlchemy
from sqlalchemy import create_engine, Column, Integer, String from sqlalchemy.ext.declarative import declarative_base from sqlalchemy.orm import sessionmaker engine = create_engine('sqlite:///users.db') Base = declarative_base() class User(Base): __tablename__ = 'users' id = Column(Integer, primary_key=True) name = Column(String) Base.metadata.create_all(engine) Session = sessionmaker(bind=engine) session = Session() new_user = User(name='Bob') session.add(new_user) session.commit() users = session.query(User).all()
14. Testing and Debugging
Unit Testing with unittest
import unittest def add(a, b): return a + b class TestAdd(unittest.TestCase): def test_add(self): self.assertEqual(add(2, 3), 5) if __name__ == '__main__': unittest.main()
Debugging with pdb
import pdb pdb.set_trace() # Your code here
Logging
import logging logging.basicConfig(level=logging.INFO) logging.info('This is an info message')
15. Deployment and Version Control
Using Git
- Initialize Repository:
git init
- Add and Commit:
git add . git commit -m "Initial commit"
- Push to GitHub:
git remote add origin https://github.com/username/repo.git git push -u origin master
Deploying to Heroku
- Install Heroku CLI: devcenter.heroku.com/articles/heroku-cli
- Commands:
heroku login heroku create appname git push heroku master
16. Best Practices and Coding Conventions
PEP 8 Guidelines
- Indentation: 4 spaces per level.
- Maximum Line Length: 79 characters.
- Naming Conventions:
- Variables and functions:
lower_case_with_underscores
- Classes:
CapWords
- Constants:
ALL_CAPS
- Variables and functions:
Writing Clean Code
- Modular Code: Break code into reusable functions and classes.
- Readability: Use meaningful variable and function names.
- Documentation: Include docstrings and comments.
17. Practical Projects
Project Ideas
- Calculator Application
- To-Do List Manager
- Web Scraper
- Simple Web Blog
Getting Started
- Plan the Project: Outline features and functionalities.
- Set Up Environment: Create virtual environment and initialize version control.
- Iterative Development: Build and test small components.
18. Additional Resources
Learning Platforms
- Official Documentation: docs.python.org
- Tutorials: Real Python, Programiz
- Online Courses: Coursera, Udemy, edX
Community and Support
- Forums: Stack Overflow, Reddit r/Python
- Local Meetups: Look for Python user groups in your area.
Useful Code Snippets
Swap Variables
a, b = b, a
List Flattening
flat_list = [item for sublist in nested_list for item in sublist]
Check for Even Numbers in a List
evens = [num for num in numbers if num % 2 == 0]
Dictionary Comprehension
squared_numbers = {x: x**2 for x in range(5)}
Enumerate in Loops
for index, value in enumerate(my_list): print(f"Index: {index}, Value: {value}")
Remember, this cheatsheet is a quick reference. For in-depth understanding, experiment with the code and refer to official documentation and comprehensive tutorials.
See why AskCodi beats other AI assistants every time.
Discover all the amazing things you'll create with AI‑powered coding assistance.
🌐Works with 65+ languages
⚡Real‑time inline suggestions
⭐Multiple top‑tier AI models